namespace Microsoft.FSharp
type Users = obj
Full name: typeproviders.Users
Multiple items
val string : value:'T -> string
Full name: Microsoft.FSharp.Core.Operators.string
--------------------
type string = System.String
Full name: Microsoft.FSharp.Core.string
namespace Microsoft.FSharp.Data
Multiple items
type LiteralAttribute =
inherit Attribute
new : unit -> LiteralAttribute
Full name: Microsoft.FSharp.Core.LiteralAttribute
--------------------
new : unit -> LiteralAttribute
val printfn : format:Printf.TextWriterFormat<'T> -> 'T
Full name: Microsoft.FSharp.Core.ExtraTopLevelOperators.printfn
val box : value:'T -> obj
Full name: Microsoft.FSharp.Core.Operators.box
Multiple items
val int : value:'T -> int (requires member op_Explicit)
Full name: Microsoft.FSharp.Core.Operators.int
--------------------
type int = int32
Full name: Microsoft.FSharp.Core.int
--------------------
type int<'Measure> = int
Full name: Microsoft.FSharp.Core.int<_>
Multiple items
val float : value:'T -> float (requires member op_Explicit)
Full name: Microsoft.FSharp.Core.Operators.float
--------------------
type float = System.Double
Full name: Microsoft.FSharp.Core.float
--------------------
type float<'Measure> = float
Full name: Microsoft.FSharp.Core.float<_>
F# Type Providers
The Current State
What are Type Providers?
(In F# since v3.0, August 2012)
Classic TP intro from Don Syme
- The world is information-rich
- Modern applications are information-rich
- Our languages are information-sparse
This is a problem!
CodeGen - the classic approach
WCF |
=>
|
svcutil.exe |
=>
|
T1 |
SQL DB |
=>
|
edmgen.exe |
=>
|
T2 |
OData |
=>
|
datrasvcutil.exe |
=>
|
T3 |
A Type Provider is ...
... a component that provides types, properties,
and methods for use in your program.
As well as a compiler/IDE extension…
JSON Type Provider
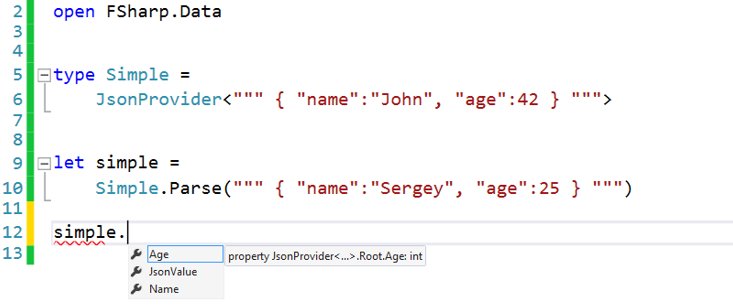
Which Type Provides exist?
F# 3.0 Built-in Type Provides
- WsdlService
- EdmxFile
- ODataService
- DbmlFile (DBML file based)
- SqlDataConnection (LINQ to SQL)
- SqlEntityConnection (LINQ to Entities)
- CSV Type Provider
- HTML Type Provider
- JSON Type Provider
- XML Type Provider
- WorldBank Provider
- Freebase Provider
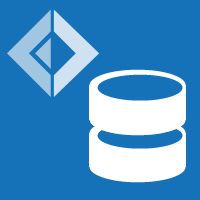
WorldBank Type Provider
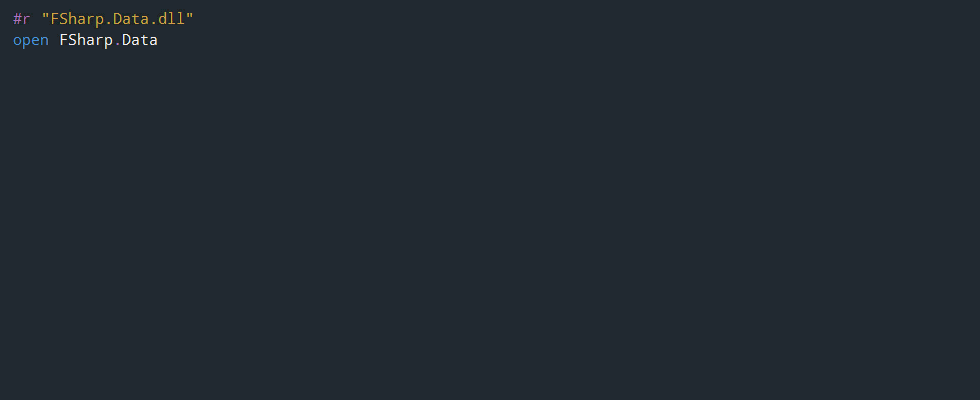
- FileSystem
- Registry
- WMI
- PowerShell
- SystemTimeZonesProvider
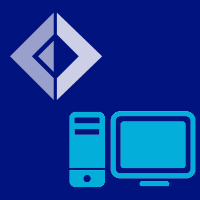
FileSystem Type Provider
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
|
// reference the type provider dll
#r "FSharp.Management.dll"
open FSharp.Management
// Let the type provider do its work
type Users = FileSystem<"C:\\Users\\">
// now you have typed access to your filesystem
// and you can browse it via Intellisense
Users.AllUsers.Path
val it : string = "C:\Users\All Users"
|
PowerShell Type Provider
1:
2:
3:
4:
5:
|
#r "System.Management.Automation.dll"
#r "FSharp.Management.PowerShell.dll"
open FSharp.Management
type PS = PowerShellProvider< "Microsoft.PowerShell.Management" >
|
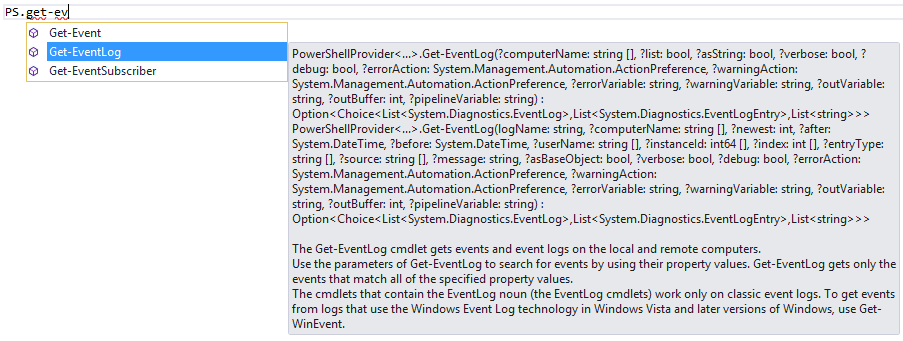
- SqlCommandProvider - type-safe access to full set of T-SQL language
- SqlProgrammabilityProvider - quick access to Sql Server functions, stored procedures and tables in idiomatic F# way
- SqlEnumProvider - generates enumeration types based on static lookup data from any ADO.NET complaint source
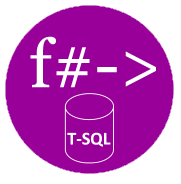
SqlCommandProvider
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
|
open FSharp.Data
[<Literal>]
let connectionString =
@"Data Source=.;Initial Catalog=AdventureWorks2014;Integrated Security=True"
do
use cmd = new SqlCommandProvider<"
SELECT TOP(@topN) FirstName, LastName, SalesYTD
FROM Sales.vSalesPerson
WHERE CountryRegionName = @regionName AND SalesYTD > @salesMoreThan
ORDER BY SalesYTD
" , connectionString>()
cmd.Execute(topN=3L, regionName="United States", salesMoreThan=1000000M)
|> printfn "%A"
|
R Provider
The F# Type Provider is a mechanism that enables smooth interoperability
between F#
and R
. The Type Provider discovers R packages that are
available in your R installation and makes them available as .NET
namespaces underneath the parent namespace RProvider
.
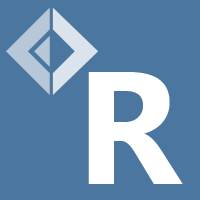
R Provider : Linear regression
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
17:
18:
19:
20:
|
// Random number generator
let rng = Random()
let rand () = rng.NextDouble()
// Generate fake X1 and X2
let X1s = [ for i in 0 .. 9 -> 10. * rand () ]
let X2s = [ for i in 0 .. 9 -> 5. * rand () ]
// Build Ys, following the "true" model
let Ys = [ for i in 0 .. 9 ->
5. + 3. * X1s.[i] - 2. * X2s.[i] + rand () ]
let dataset =
namedParams [
"Y", box Ys;
"X1", box X1s;
"X2", box X2s; ]
|> R.data_frame
let result = R.lm(formula = "Y~X1+X2", data = dataset)
|
FSharp.Configuration
- AppSettings
- ResX
- Yaml
- Ini
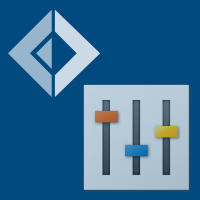
Yaml Type Provider
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
|
Mail:
Smtp:
Host: smtp.sample.com
Port: 25
User: user1
Password: pass1
DB:
ConnectionString: ...
NumberOfDeadlockRepeats: 5
DefaultTimeout: 00:05:00
|
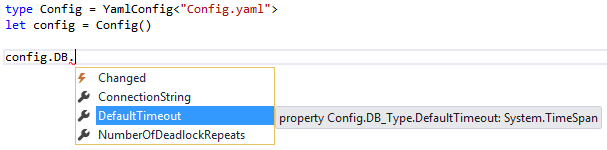
What else exist
- Apiary
- Freebase
- Excel
- Graph
- Math
- Xaml
- CRM
- DbPedia
- Twitter
- RSS
- NuGet
- DGML
- DataStore
- Hadoop/Hive/Hdfs
- MiniCvs
- COM
- FunScript
- Matlab
- IKVM
- Python
- Azure
- S3
- Neo4j
- Swagger (WIP)
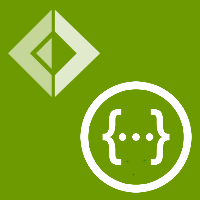
Welcome to join development
Swagger Sample (WIP)
1:
2:
3:
4:
5:
6:
|
#r "SwaggerProvider.dll"
open SwaggerProvider
[<Literal>]
let apiSchemaUrl = "http://petstore.swagger.io/v2/swagger.json"
let PetStore = SwaggerProvider<apiSchemaUrl>
|
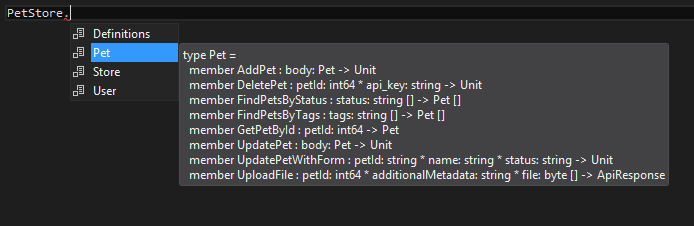
F# Code Quotations : Basics
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
|
let a = 42
//val a : int = 42
let b = <@ 42 @>
//val b : Quotations.Expr<int> = Value (42)
let c = <@@ 42 @@>
//val c : Quotations.Expr = Value (42)
let d = <@ 2+2 @>
//val d : Quotations.Expr<int> =
// Call (None, op_Multiply, [Value (2), Value (2)])
|
F# Code Quotations
1:
2:
3:
4:
5:
6:
|
let f =
<@ let rec g x =
match x with
| 0 -> 1
| _ -> x * g(x-1)
g 5@>
|
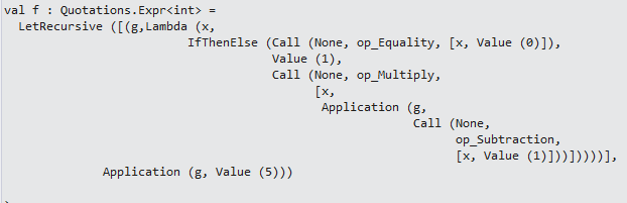
F# Code Quotations : Splicing
1:
2:
3:
4:
5:
6:
7:
8:
|
let f3 n =
<@ let rec g = function
| 0 -> 1
| x -> x * g(x-1)
g %n@>
let combined_quotation =
f3 <@ 3 @>
|
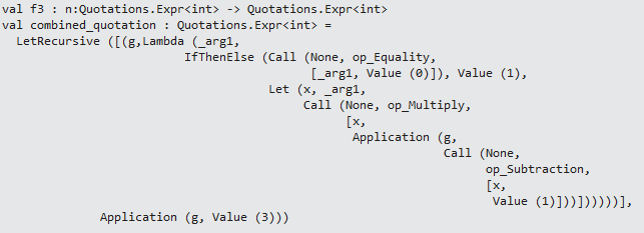
Custom Zip TP
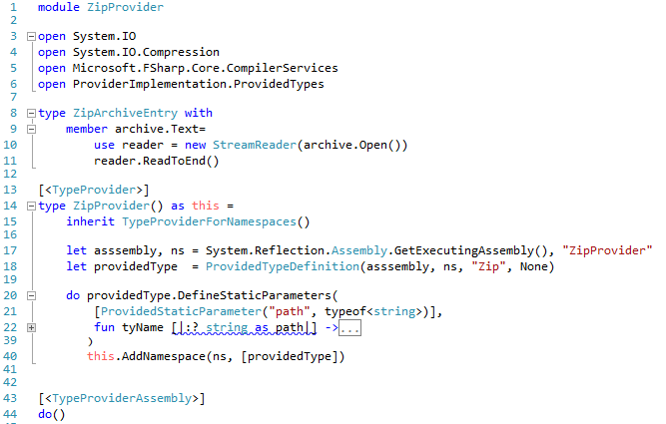
Custom Zip TP
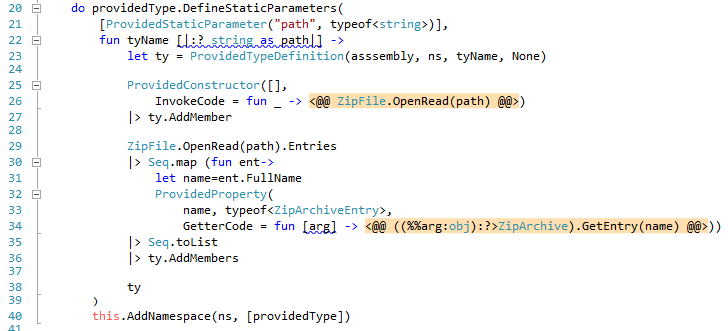
Zip TP Sample
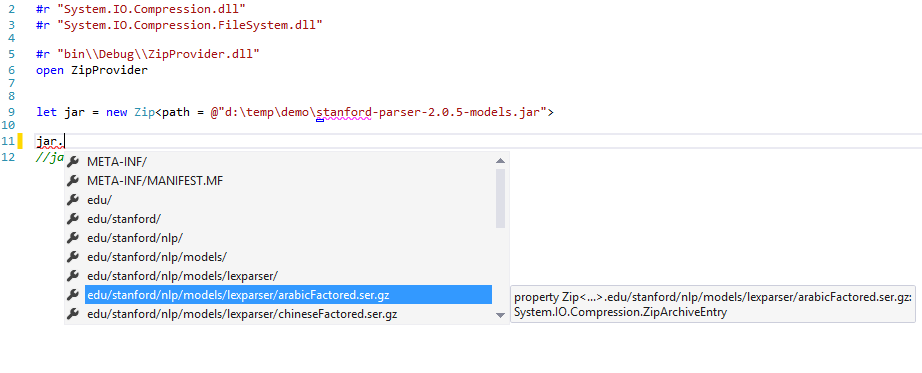
Generated vs erased type providers
Support for static parametes to
provided methods
1:
2:
|
ProvidedType.ProvidedMethod<staticParam>
(providedParam1,..., providedParamM) -> returnType
|
-
Provided method can change its number of parameters with the static parameter
given to the method.
-
The return type can likewise be changed (and can be a
provided type which name depends on the input parameter)
1:
2:
3:
4:
5:
|
let x = ExampleType()
let v1:int = x.Average<1>(1)
let v2:float = x.Average<2>(1,2)
let v3:float = x.Average<3>(1,2,3)
|
Opportunity #1 : Regular Expressions
1:
2:
|
let [<Literal>] phonePattern =
@"^(?:(?<AreaCode>\d{3})-)?(?<PhoneNumber>\d{3}-\d{4})$"
|
Traditional usage
1:
2:
3:
|
type PhoneReg = RegexTyped<phonePattern>
let phone = PhoneReg()
phone.Match("800-374-2774").PhoneNumber
|
Opportunity #2 : SafeString
A simple example is a provided safe string type which enforces that
the number of slots in a String.Format
format string matches the number
of arguments passed.
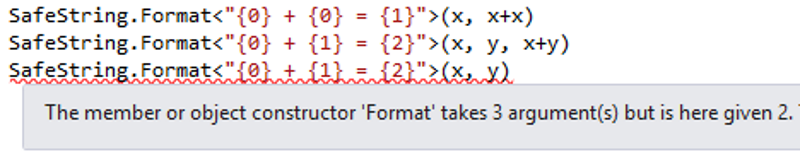
Sample from Announcement of F# 4.0 preview
Opportunity #3 : SqlCommandProvider
SqlCommandProvider from FSharp.Data.SqlClient
1:
2:
|
type MyCommand1 = SqlCommandProvider<"SELECT 42", connStr>
type MyCommand2 = SqlCommandProvider<"SELECT 43", connStr>
|
can be rewritten as
1:
2:
3:
4:
|
type MyDb = SqlCommandProvider<connStr>
type MyCommand1 = MyDb.GetCommand<"SELECT 42">
type MyCommand2 = MyDb.GetCommand<"SELECT 43">
|
Benefits:
- Shared DB definition
- Shared provided types
Sample provided by Dmitry Morozov
Opportunity #4: CSV Data manipulation
A modified CSV type provider that lets you add a column.
1:
2:
3:
4:
5:
6:
7:
|
type MyCsvFile = FSharp.Data.CsvProvider<"mycsv.csv">
let csvData = MyCsvFile.LoadSample()
[ for row in csvData -> row.Column1, row.Column2 ]
let newCsvData = csvData.WithColumn<"Column3", "int">()
[ for row in newCsvData -> row.Column1, row.Column2, row.Column3 ]
|
Don Syme's sample from SampleMethStaticParamProvider
Opportunity #5: Search in the
Freebase or DbPedia provider
1:
2:
3:
4:
5:
|
type DbPedia = DbPediaProvider<"Some Parameters">
let ctxt = DbPedia.GetDataContext()
let princeTheMusician = ctxt.Ontology.People.Search<"Prince">.
|
In the intellisense at the last point the completions for all people matching "Prince" would be shown
Don Syme's sample from SampleMethStaticParamProvider
Opportunity #6
Any your ...
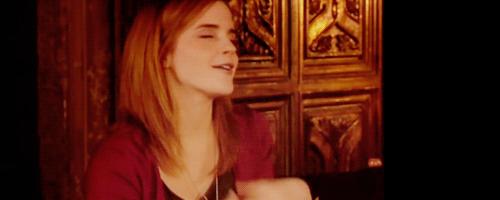
... idea